Debugger
Debugging program code is the process of trouble-shooting program statements to fix either syntax or semantic errors in your program. Modern programming environments have lots of tools to identify syntax errors for programmers and debugging tools to help find and fix semantic or logic errors.
Syntax errors are the things like mispelled command or variable names, incorrect statement punctuation, forgetting required inputs, including the wrong number, type or order of arguments to a function call, etc.
Semantic errors are also known as logic errors. It is rarely possible for computer programs to identify semantic errors. These types of errors occur when the program does something, but it is not what the programmer intended or needs. It is up to the programmer to understand what the program is supposed to do and whether or not it performs correctly.
Debugging tools have been available all along and now we present some of them. This screen shot shows a typical editor window with the debugging buttons circled. Notice how the tools are available when you edit your program code from Matlab's Editor. The tools are not available when editing program files in using other file editors.
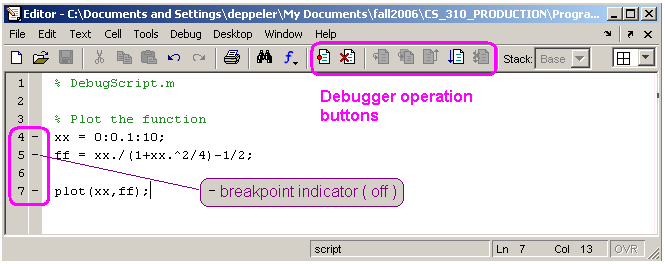
Program Breakpoint
Think of a breakpoint as a stop sign in your program. The debugger reaches that line in the code and stops executing code until you give it the okay (command) to continue. Multiple breakpoints are like multiple stop signs, each time one breakpoint is reached, execution stops allowing the programmer to inspect variables before continuing with the program's execution. Here is what a script looks like with one breakpoint set.
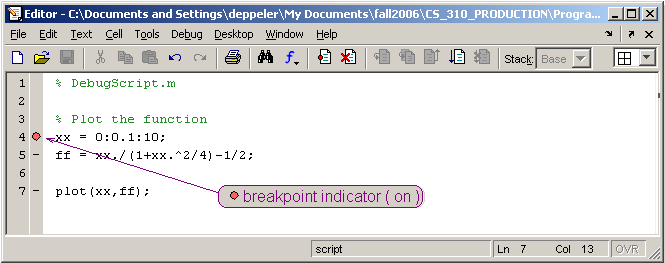
When a program with one or more breakpoints is run, Matlab activates several useful buttons for common debugging operations. This screen shot shows the available options once a program is started in debug mode.
![screen shot with debug buttons [Step] [Step in] [Step out] [Continue] [Exit debug mode] circled](debug_screen.png)
Why set a breakpoint?
To have the option of executing any number of debugging operations before continuing with the program's execution. Once execution has stopped, the programmer can examine or change the values of any variables, and continue by executing the code one line at a time, step in in to a function (to execute that function's code one line at a time), continue executing to the next breakpoint, quit the debugger. Here's a table that lists the common debugging operations.
Button | Operation | Description |
---|---|---|
![]() | Step | execute the current line and stop at the next line |
![]() | Step in | enter the function and execute the first line of the functioni and stop at the next line |
![]() | Step out | execute the remaining lines of the current function and return to the line after the line that called the function. |
![]() | Continue | continue executing all lines of code until the next breakpoint |
![]() | Exit debug mode | stop executing the program in debug mode |
It takes a fair amount of programming experience to become an efficient code debugger. The debugger operations help programmers get very detailed information about a few lines of code at a time. However, stepping through code is a very time consuming process. Incremental development -- designing, coding, and testing a few lines of code at a time, before adding more code -- is an effective programming technique for reducing bugs in the first place which reduces the need for debugging.
Here are still other common and easy to use MATLAB debugging techniques:
- OUTPUT results of each line.
Remove the semi-colons (
;
) from selected lines of code to see the output when the program is run. This can help you understand how the program is executing (e.g. the number of times a loop is running) and see what values are being assigned at various places in the program. - COMMENT OUT lines or blocks of code that aren't working and add them in one or a few line(s) at a time.
Select the lines you wish to ignore for now.
Right-click and select "Comment" or use [Ctrl-R] to comment out the selected lines.
By placing the comment character in front of statements you temporarily don't want to run, you may be able to more quickly pinpoint problems in other lines of code.
- Use the MATLAB text editor to quickly comment out and uncomment large portions of text for you - Highlight the text and choose the Text->Comment or Text->Uncomment menu items.